Creating Web Ads¶
Static Web Ads¶
To create a static web ad, you need to specify:
Advertisable EID to add the advertisement to
Destination URL where to send the user when the ad is clicked
Image file to upload
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
-F advertisable=8597013E81481B0FE28772 \
-F name="Test Ad 300x250" \
-F destination_url=https://developers.nextroll.com \
-F file=@300x250.png \
https://services.adroll.com/api/v1/ad/create?apikey=MYAPIKEY
Response:
{
"results": {
"eid": "2A842B75A34EF695A5AA9D",
"name": "Test Ad 300x250",
// ...
}
}
Native Ads¶
To create Native Ads, you’ll need to specify type=native
and inventory_type=iab
when calling POST /api/v1/ad/create
. For the list of supported image sizes, please refer to the Ad Format Guidelines.
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
-F advertisable=8597013E81481B0FE28772 \
-F type=native \
-F inventory_type=iab \
-F name="Test Native Ad" \
-F body="This is the description" \
-F destination_url=https://www.example.com/ \
-F file=@600x500.png \
'https://services.adroll.com/api/v1/ad/create?apikey=MYAPIKEY'
Response:
{
"results": {
"eid": "EABF2CB2DEED8B777E8306",
"name": "Test Native Ad",
"type": "native",
// ...
}
}
Dynamic Native Ads¶
To create Dynamic Native Ads, you’ll need to specify several parameters:
advertisable
Advertisable EID to create the native ad in
name
Name of the ad when shown in the dashboard and in reports
type
Always
native
inventory_type
Always
iab
is_liquid
Always
true
format
Always
33
(600x315)headline
Called Title in the dashboard. When rendered, the value may be truncated after 25 characters.
message
Called Description in the dashboard. When rendered, the value may be truncated after 125 characters.
brand_name
Called Brand Name in the dashboard. When rendered, the value may be truncated after 30 characters.
destination_url
Fallback destination URL. When the ad is rendered, the product’s URL will be used.
file
Fallback image. When the ad is rendered, the product’s image will be used. Pass a base64-encoded string or use the multipart/form-data content-type.
The headline
, message
, and brand_name
parameters support the following macros that pull values from your product feed. These macros must be mapped to fields in the product feed to be useable.
%%title%%
Title of the product
%%description%%
Description of the product
%%price%%
Price of the product
%%brand%%
Brand name of the product
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
-F advertisable=8597013E81481B0FE28772 \
-F type=native \
-F inventory_type=iab \
-F is_liquid=true \
-F name="Test Dynamic Native Ad" \
-F headline="%%title%%" \
-F message="%%description%%" \
-F brand_name="%%brand%%" \
-F destination_url=https://www.example.com/ \
-F file=@600x315.png \
'https://services.adroll.com/api/v1/ad/create?apikey=MYAPIKEY'
Response:
{
"results": {
"eid": "EABF2CB2DEED8B777E8306",
"name": "Test Native Ad",
"type": "native",
// ...
}
}
Dynamic Web Ads¶
To create Dynamic Web Ads, you first need to ensure you’ve uploaded a logo for your Advertisable.
Upload a Logo¶
You can upload a logo for an advertisable by calling /api/v1/advertisable_logo/create
. You only need to do this once or if you want to change the logo.
The endpoint expects the logo to be passed as a base64-encoded data. Here’s an example of base64-encoding an image on the command line using Python:
python -m base64 < 320x50.png > 320x50.png.txt
Request:
This example uses the name@filename
syntax for the --data-urlencode
cURL parameter which will URL-encode the contents of the file before sending it.
curl -H 'Authorization: Token YOUR_TOKEN' \
-d advertisable=8597013E81481B0FE28772 \
--data-urlencode logo_file@320x50.png.txt \
https://services.adroll.com/api/v1/advertisable_logo/create?apikey=MYAPIKEY
Response:
{
"results": {
"id": 1234,
"advertisable_id": 1234,
"s3_logo_path": "https://s.adroll.com/logos/8597013E81481B0FE28772/CS5ETCEWB5GQRHCJ3ANOG5",
"width": 200,
"height": 31
}
}
Create the Dynamic Web Ads¶
You create a set of Dynamic Web Ads by calling /api/v1/ad/create_templated_web_ads
. When calling the method, you need to specify the ID of the template you’d like to use. The table below provides the IDs and examples. The endpoint returns a list of ads created, which you can then add to your campaigns.
Template ID |
Theme name |
---|---|
0 |
|
1 |
|
2 |
|
3 |
|
4 |
|
10 |
|
13 |
|
21 |
|
22 |
|
28 |
|
29 |
|
33 |
|
36 |
|
45 |
|
47 |
|
64 |
|
72 |
|
80 |
|
83 |
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
-d advertisable=8597013E81481B0FE28772 \
-d name='test' \
-d theme_color='#FFFFFF' \
-d text_cta='Shop Now' \
-d dynamic_template_id=1 \
https://services.adroll.com/api/v1/ad/create_templated_web_ads?apikey=MYAPIKEY
Response:
{
"results": [
{
"eid": "05995D19B6B19DD08AAF00",
"name": "Dynamic_728x90_3/3/2017_1_test",
// ...
}
]
}
Retrieve List of Dynamic Web Templates¶
Call GET /api/v1/dynamic_template/get_all_for_advertisable
to retrieve the list of available templates. To create ads, use the value of the id
field from the template as the dynamic_tempalte_id
parameter in POST /api/v1/ad/create_templated_web_ads
. See Create the Dynamic Web Ads for more on creating ads.
The response includes a list of capabilities. You can use the capabilities
list to decide what fields to prompt the user for. For example, if you see the salePrice
capability, then you’ll know you can use the sale_price_option
field when calling POST /api/v1/ad/create_templated_web_ads
. Note that capabilities are optional.
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
'https://services.adroll.com/api/v1/dynamic_template/get_all_for_advertisable?advertisable=8597013E81481B0FE28772&apikey=MYAPIKEY'
Response:
{
"results": [
{
"id": 33,
"eid": "6SJ2LVKKLZHNFCBGRZLPXA",
"category": null,
"in_testing": false,
"is_private": false,
"is_published": true,
"is_visible": true,
"name": "Holiday Bow NEW!",
"capabilities": [
{
"id": 2,
"eid": "RJTMLC5L4ZDVFJSGV3EMOJ",
"name": "salePrice",
"description": "Sale price",
"is_enabled": true,
"type": null
}
],
"allowed_advertisables": []
}
]
}
Retrieve List of Dynamic Web Templates Capabilities¶
Call GET /api/v1/dynamic_template_capability_description/get_all
to query the full list of capabilities that might appear in templates.
Request:
curl -H 'Authorization: Token YOUR_TOKEN' \
'https://services.adroll.com/api/v1/dynamic_template_capability_description/get_all?apikey=MYAPIKEY'
Response:
{
"results": [{
"id": 1,
"eid": "CHU4RLZSVJCVNG6ZELX2RM",
"name": "promoText",
"description": "Promo text",
"is_enabled": true,
"type": null
}, {
"id": 2,
"eid": "RJTMLC5L4ZDVFJSGV3EMOJ",
"name": "salePrice",
"description": "Sale price",
"is_enabled": true,
"type": null
}]
}
Dynamic Web Templates¶
Classic Spotlight¶
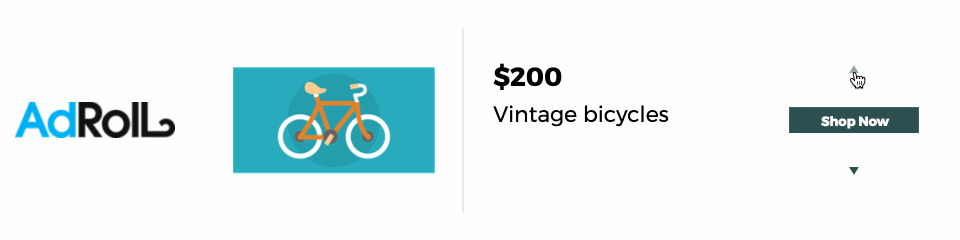
Classic carousel¶
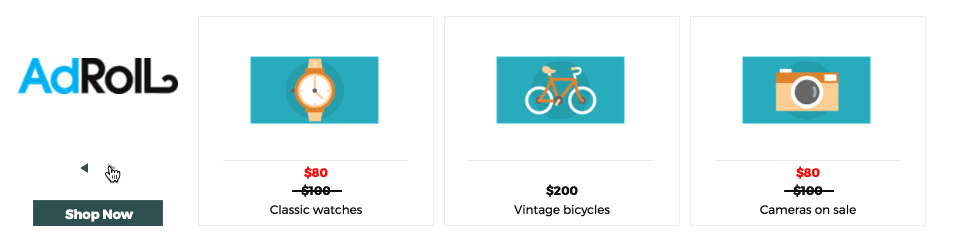
Duotone carousel¶
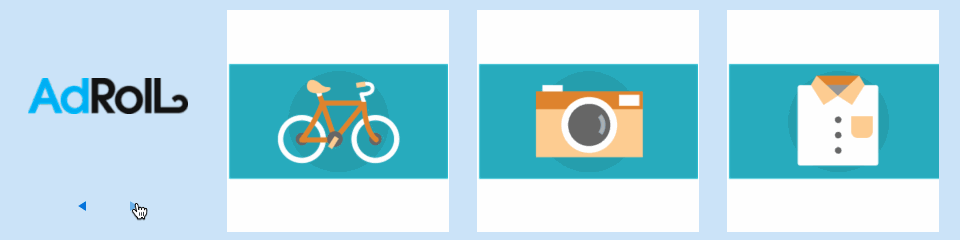
Holiday spotlight¶
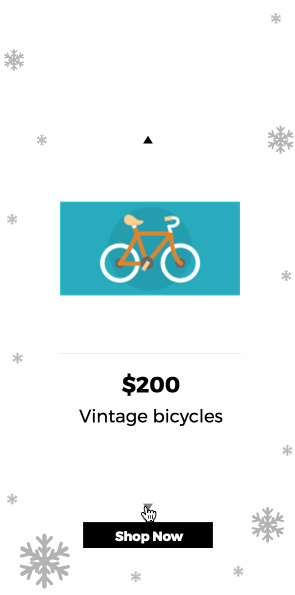
Holiday carousel¶
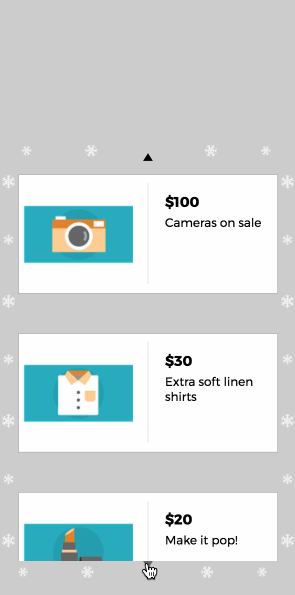
Valentine carousel¶
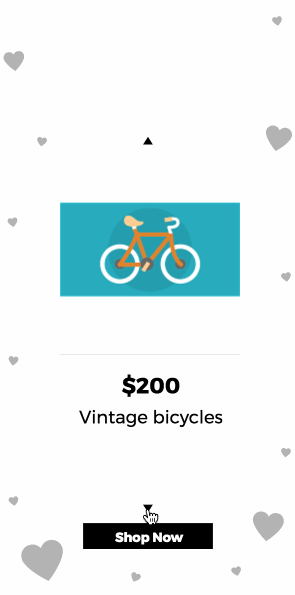
Valentine spotlight¶
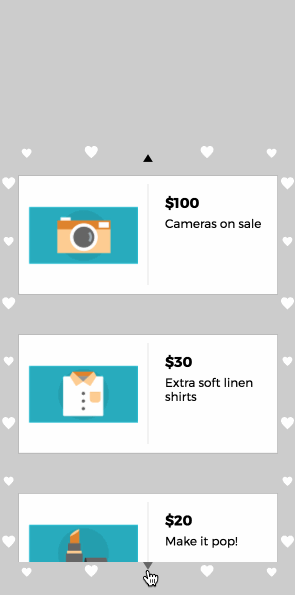
Fall carousel¶
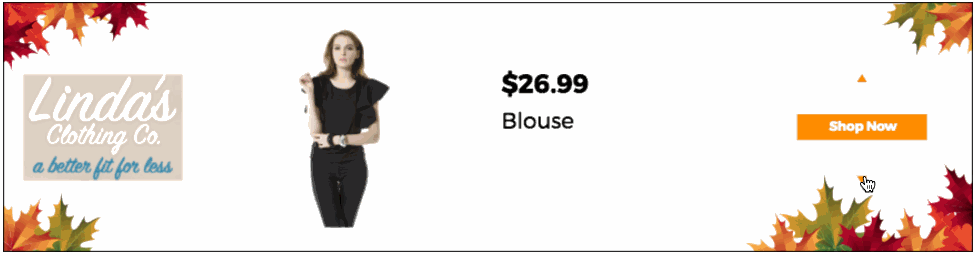
Fall spotlight¶
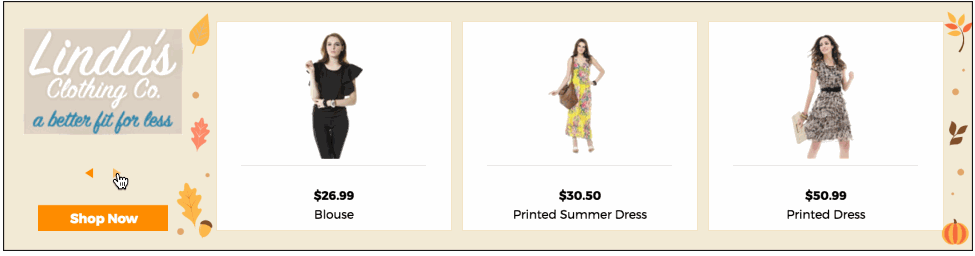
Black Friday carousel¶
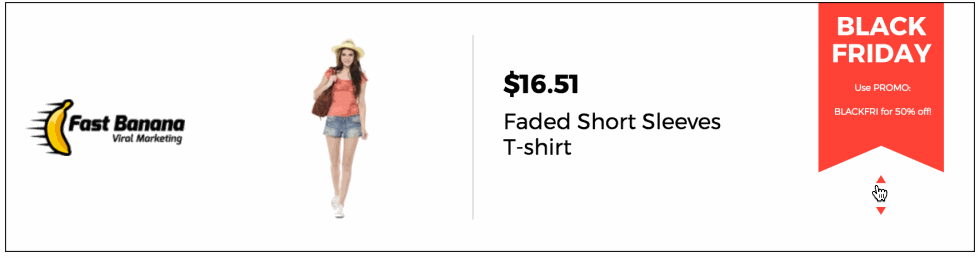
Cyber Monday carousel¶
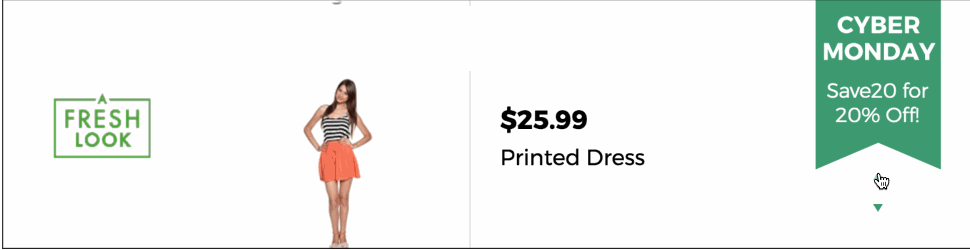
Holiday Bow carousel¶
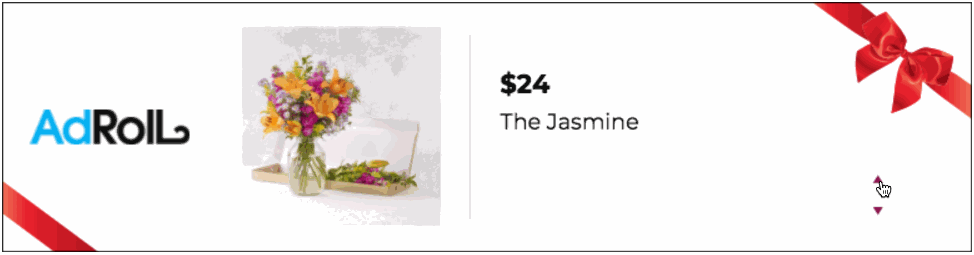
Holiday Sparkle carousel¶
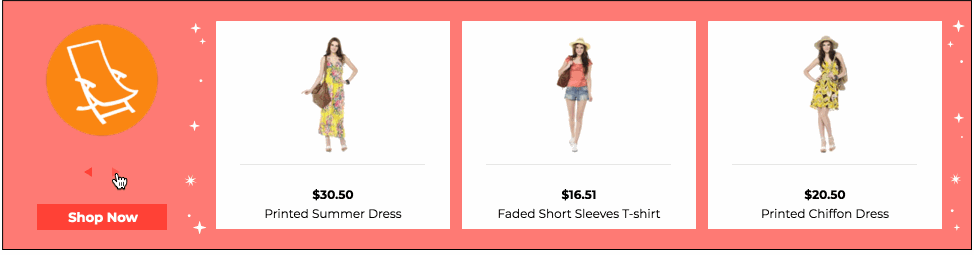
Valentine 2018 spotlight¶
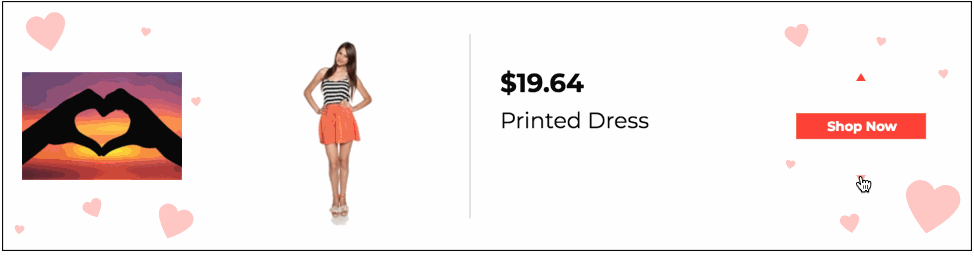
Valentine 2018 carousel¶
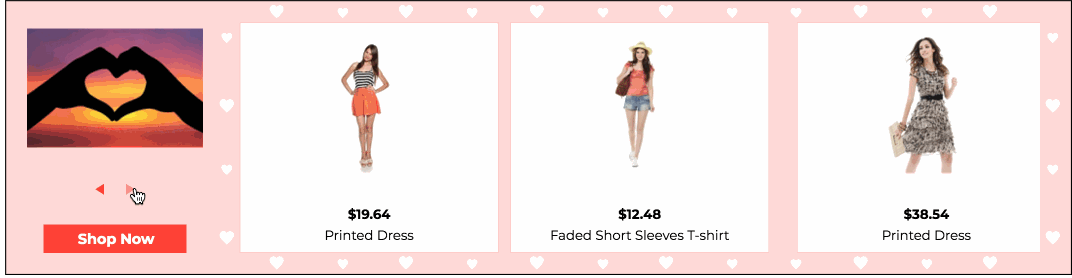
Product Gladiator¶
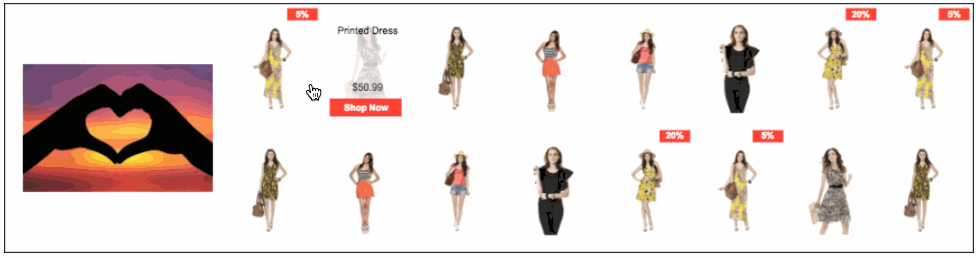
Spring Stripes¶
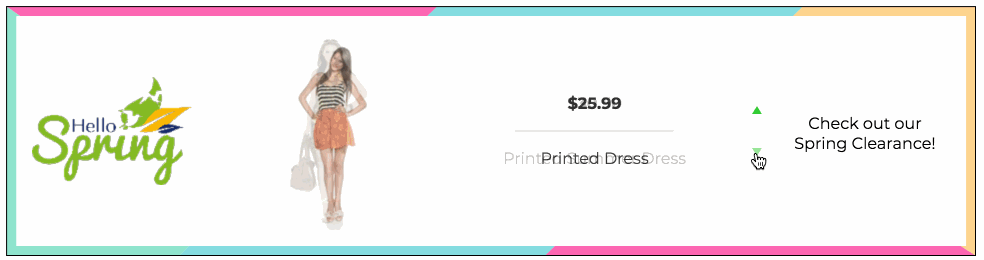
Summer Polka Dots¶
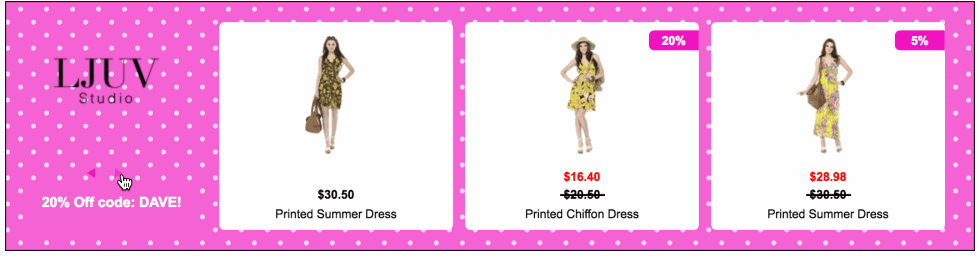
Summer Beach¶
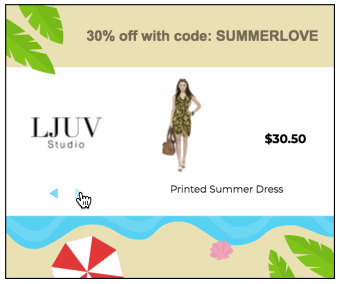